Creating a QR code is a simple, do-it-yourself project that can be accomplished with a variety of tools, including mobile apps, web browsers, and dedicated QR code generation websites. QR codes can be used for a wide range of purposes, from linking to a personal website or digital business card, to simplifying the process of connecting to a WiFi network. Below, I’ll guide you through the process of generating your own QR code using different methods.
Contents
Using Mobile Phone
Generating QR Codes on Safari for iPhone
Creating QR codes directly from Safari on an iPhone offers a seamless way to share webpages without needing to switch to a dedicated app. Although Safari does not natively support QR code generation, the iOS Shortcuts app provides a powerful workaround:
- Open the Shortcuts App: Locate and open the Shortcuts app on your iPhone.
- Create a New Shortcut: Tap the “+” sign to start a new shortcut.
- Add the QR Code Generation Action: Search for “QR” and select “Generate QR Code” from the list of actions.
- Configure the Shortcut: The default setting uses the clipboard for input, making it perfect for use with Safari. Simply copy any URL from Safari to generate its QR code.
- Save and Name Your Shortcut: Finish by naming your shortcut, such as “Generate QR Code.”
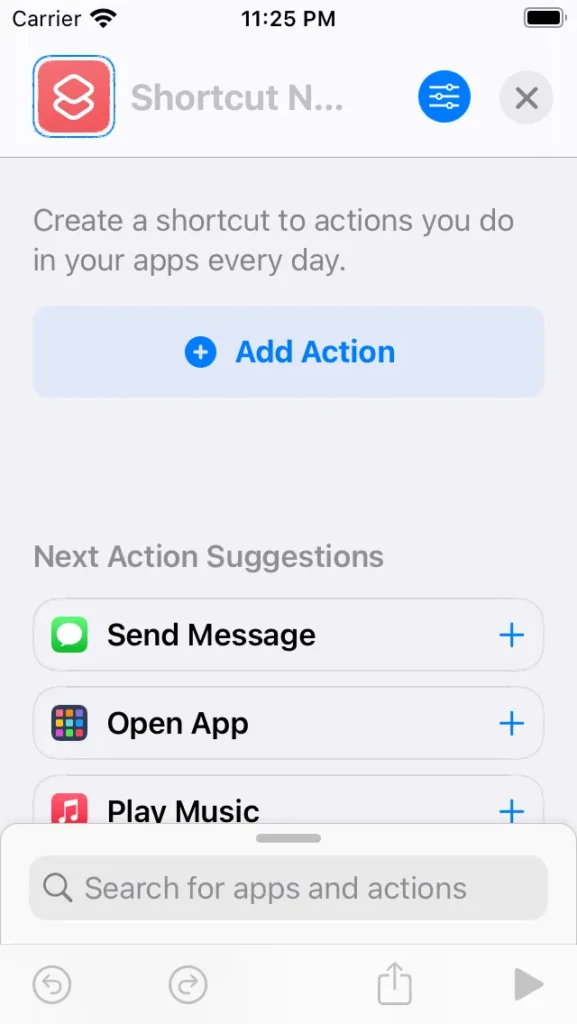
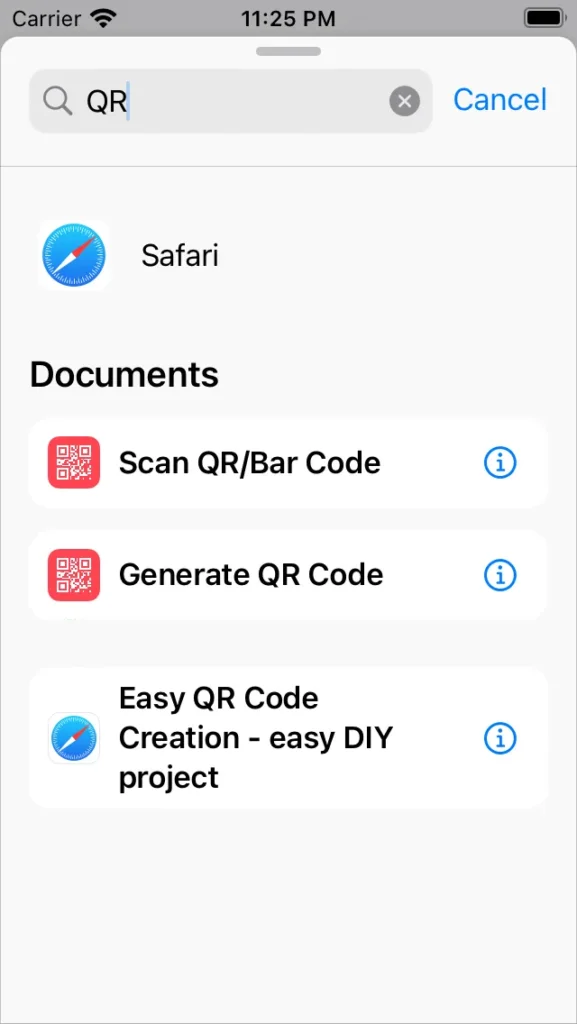
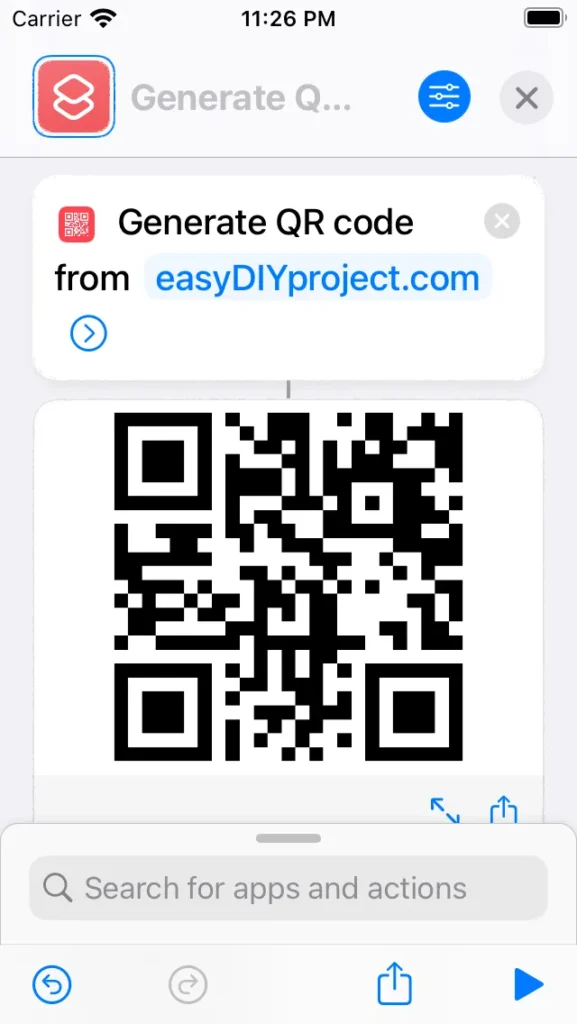
To use the shortcut, copy a URL from Safari, then run the shortcut to generate a QR code, which can be saved or shared directly from your iPhone.
Generating QR Codes on Chrome for Android
Google Chrome on Android devices simplifies the QR code generation process for URLs directly within the browser:
- Navigate to Your Desired Page: Open Chrome and go to the webpage you want to share.
- Access the QR Code Feature: Tap on the address bar and look for the QR code icon. If it’s not visible, tap on the three dots to open the menu, then select the “Share” option, followed by “QR Code.”
- Generate and Share the QR Code: Chrome will display a QR code for the webpage. From here, you can download the QR code as an image or share it through various apps.
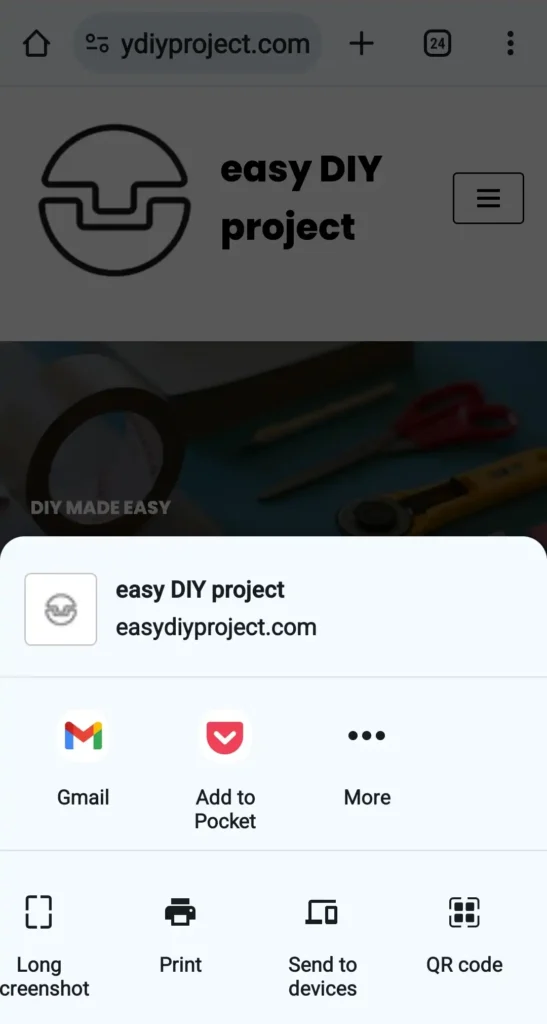
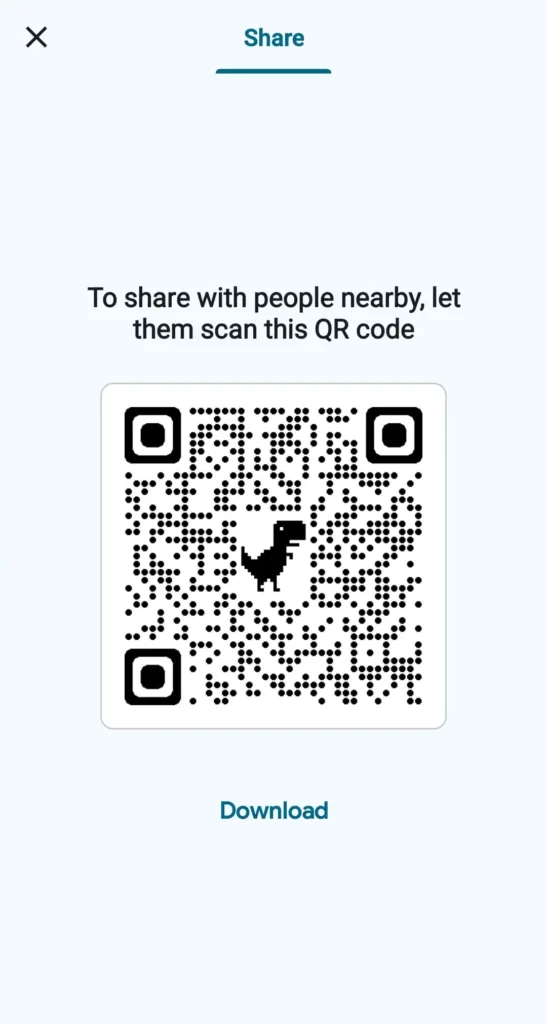
Using Chrome’s built-in feature, you can effortlessly create QR codes for any webpage, making it easier to share links in a visually appealing and accessible manner.
Using Websites
Web-based QR code generators are platform-independent and can be used on both mobile devices and computers. Here are some steps to create QR codes using these services:
- Visit a QR Code Generation Website:
- Open your web browser and go to a QR code generation site. Sites like
qr-code-generator.com
,qrcode-monkey.com
, orgoqr.me
are popular choices due to their ease of use and additional customization options.
- Open your web browser and go to a QR code generation site. Sites like
- Choose Your QR Code Content:
- Select what type of content your QR code will link to. Options typically include URL, text, email, SMS, and more.
- Enter Your Content Details:
- Fill in the specific details for your QR code, such as the URL or the text message you want to encode.
- Customize Your QR Code (Optional):
- Many sites offer customization options, allowing you to change the color, add a logo, or modify the shape of your QR code.
- Generate and Download Your QR Code:
- Click the generate button to create your QR code.
- Download the QR code image to your device for future use.
Site Links for QR Code Generation:
- QR Code Generator: https://www.qr-code-generator.com
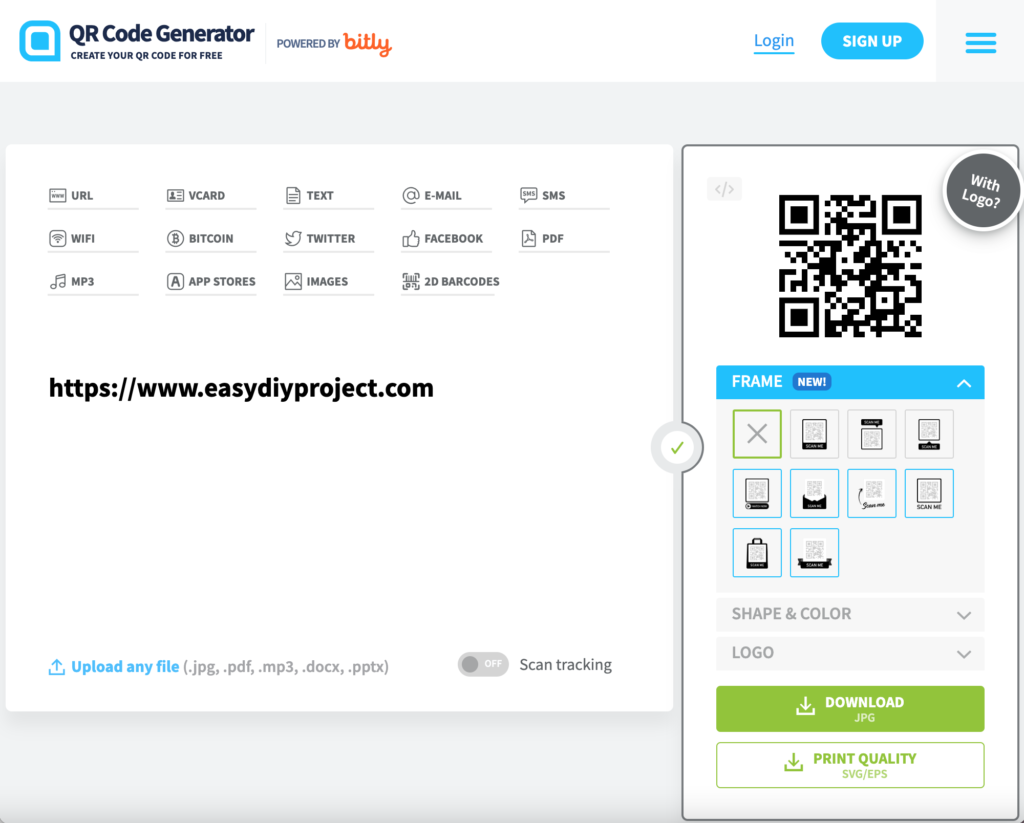
- QRCode Monkey: https://www.qrcode-monkey.com
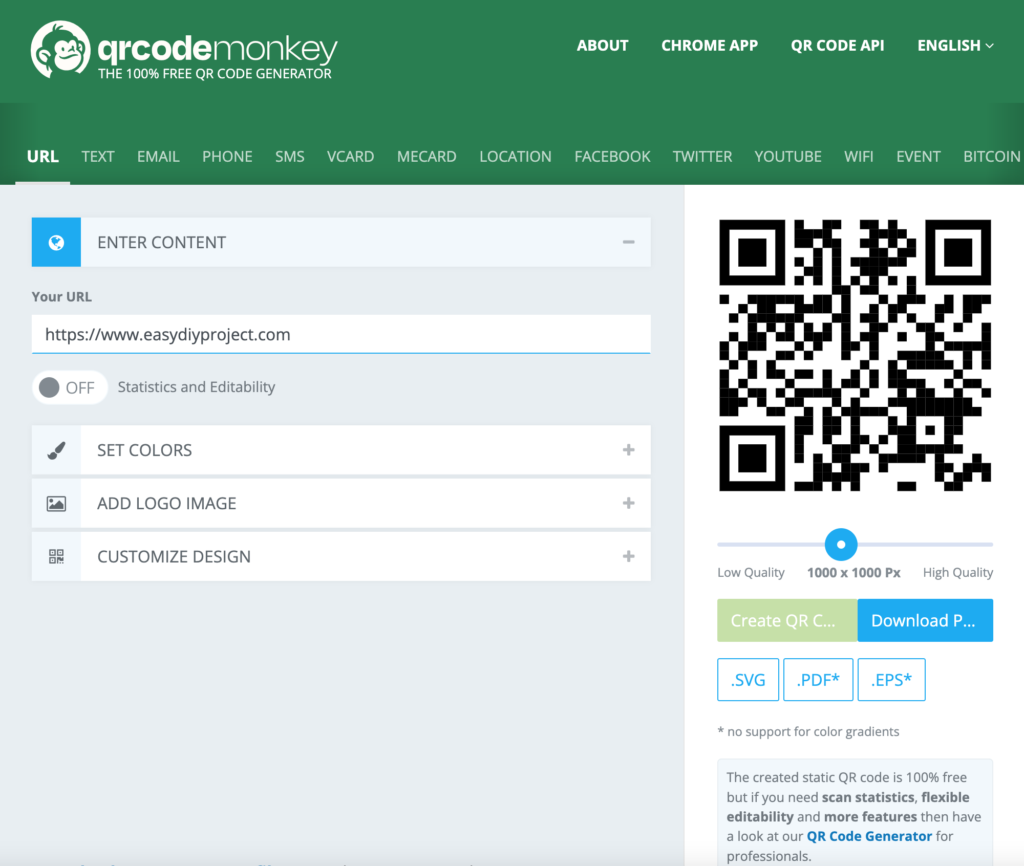
- GOQR.me: https://goqr.me
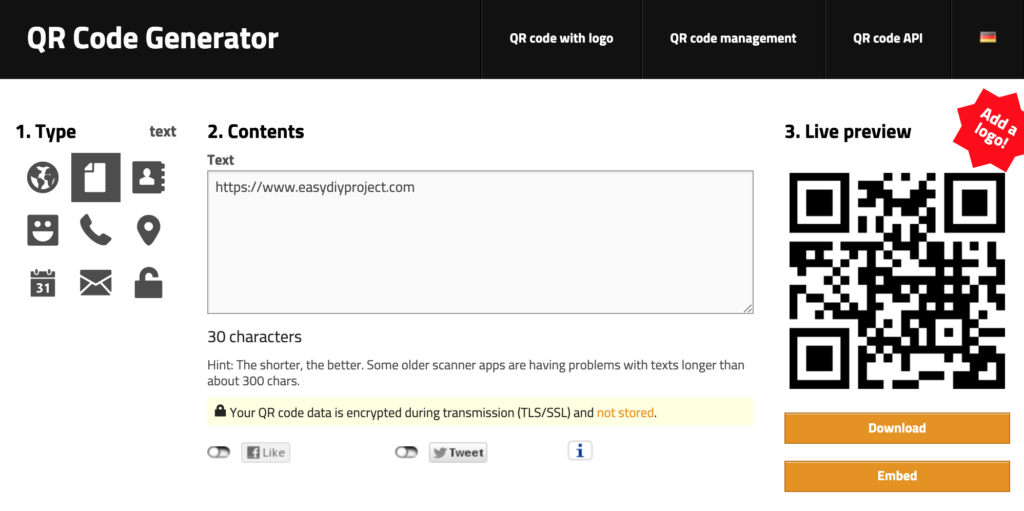
Generating QR Codes with Python
Step 1: Install the QR Code Library
First, you need to install the qrcode
library. If you haven’t installed it yet, you can do so by running the following command in your terminal or command prompt:
pip install qrcode[pil]
This command installs both qrcode
and Pillow
(through the pil
option), the latter of which is necessary for generating images.
Step 2: Write the Python Code
Next, you’ll write a Python script to generate the QR code. Here is a simple example that creates a QR code containing a URL and saves it as a PNG file:
import qrcode
# The data you want to store in the QR code (e.g., a URL)
data = "https://www.example.com"
# Create a QR code instance
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
# Add data to the instance
qr.add_data(data)
qr.make(fit=True)
# Create an Image object from the QR Code instance
img = qr.make_image(fill_color="black", back_color="white")
# Save it somewhere, change the path as needed
img.save("my_qr_code.png")
This script does the following:
- Imports the necessary library.
- Specifies the data to encode in the QR code.
- Configures the QR code’s appearance and error correction level.
- Generates an image from the QR code data.
- Saves the image as a PNG file named “my_qr_code.png”.
Step 3: Run Your Script
- Save your script with a
.py
extension, for example,generate_qr.py
. - Open your terminal or command prompt, navigate to the directory containing your script, and run it using Python:
python generate_qr.py
After running the script, you should find a new PNG image in the same directory as your script, named my_qr_code.png
. This image will contain the QR code that encodes the data you specified.
Tips for Generating QR Codes:
- Quality Matters: Ensure your QR code is clear and in a high-resolution format, especially if it will be printed.
- Test Your QR Code: Always test your QR code with multiple QR scanners to ensure it works as intended.
- Consider Your Use Case: Think about where your QR code will be displayed and how people will interact with it. This can influence the design and complexity of your QR code.
- Safety: Use reputable QR code generation services to protect your information and prevent directing users to malicious sites.